Hi guys 🙂 Varsha here and in this article we are going to discuss some basic things about C language. In this article, we will discuss loops, operators, and many other basic things in C language.
I am sure this article will definitely help you to get some brief knowledge about C language, and you can also understand the basic things about this language.
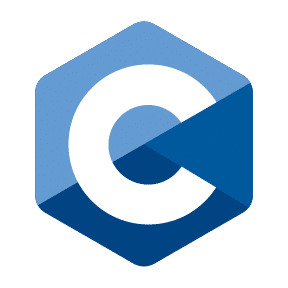
C language
C language is a programming language that is widely used for developing operating systems, games, and other applications. C is a compiled language, which means that it is converted into machine code (the language that computers can understand) by a compiler before it can be executed. A high-level language is a programming language that is closer to human language and is more abstract than a low-level language. It is easier to read, write, and understand than low-level languages, which are closer to machine code and require a deeper understanding of computer systems and hardware.
# include<stdio.h>
# include<conio.h>
void main()
{
printf("Hello World")
}
Data types in C language: –
- Integer
- Float
- Double
- Character
- Array
- String
- Pointer
- Structured
- Union
- enum
Some common knowledge about C language: –
- Token: – Token is the smallest unit of the program.
- Character cell: – integer, floating point, character, string, special character.
- Input – Output function: –
Scanf(“%d”, &n); used for input
Printf(“Hello”); used for output
- Format specifier
integer: – %d
float/double: – %f
char: – %c
alphanumeric: – %x
string: – %S
Variable scope in c language
- Local variable
- Global variable
Local variable: – if the variable is declared and called inside the function, then it is known as a local variable.
# include<stdio.h>
# include<conio.h>
void main()
{
int m = 10; // local variable
printf("%d",m);
}
Global variable: – Any variable is called a global variable if it is declared and called outside the function.
# include<stdio.h>
# include<conio.h>
int n = 20;
int main()
{
printf("%d",n);
return 0;
}
Default value: – All global variables and static, extern global variable has a default value.
# include<stdio.h>
# include<conio.h>
int num;
int main()
{
Conditional statements in c language
- if statement
- if-else statement
- if-else-if ladder
- nested if statement
if statement
Syntax:
if(condition)
{
statement
}
Example:
# include<stdio.h>
# include<conio.h>
void main()
{
int n = 10;
if(n<100)
{
printf("true")
}
}
if-else statement: –
Syntax:
if(condition)
{
statement
}
else
{
statement
}
Example:
# include<stdio.h>
# include<conio.h>
void main()
{
int n = 10;
if(n>100)
{
printf("true")
}
else
{
printf("false")
}
}
if-else-if ladder: –
Syntax:
if(condition)
{
statement
}
else if(condition)
{
statement
}
else
{
statement
}
Example:
# include<stdio.h>
# include<conio.h>
void main()
{
int n = 10, m = 20, k = 15;
if((n>m)&&(n>k))
{
printf("n is largest")
}
else if((m>n)&&(m>k))
{
printf("m is largest")
}
else
{
printf("k is largest")
}
}
Nested if statement: –
Syntax:
if(condition)
{
if(condition)
{
statement;
}
else
{
statement;
}
else
{
statement;
}
}
Example:
# include<stdio.h>
# include<conio.h>
void main()
{
int n = 10, m = 20;
if(n<50)
{
if(m<100)
{
printf("true")
}
else
{
printf("flase")
}
else
{
printf("false")
}
}
Switch Statement: –
Syntax:
switch(expression)
{
case value1: statement;
break;
case value2: statement;
break;
default: statement;
}
Example:
# include<stdio.h>
# include<conio.h>
void main()
{
int option;
printf("Enter option number:");
scanf("%d", &option);
switch(option)
{
case1: printf("runningoption-1");
break;
case2: printf("runningoption-2");
break;
case3: printf("runningoption-3");
break;
default: printf("running default");
}
}
Continue: –
Example:
# include<stdio.h>
# include<conio.h>
int main()
{
int i;
for(i=1, i<=10, i++)
{
if(i==5)
continue;
printf("%d\n",i);
}
return 0;
}
Loops in C language
In the C language, there are several types of loops that you can use to execute a block of code multiple times. These include:
- For loop
- While loop
- do while loop
- Nested for loop
For loop
this loop is often used when you want to execute a block of code a specific number of times.
Syntax:
for(initialization; condition; incre/decre)
{
statement;
}
Example:
# include<stdio.h>
# include<conio.h>
void main()
{
int i;
for (int i = 0; i < 10; i++)
{
printf("%d\n", i);
}
}
While loop
this loop continues to execute a block of code as long as a certain condition is met.
Syntax:
while(condition)
{
statement;
}
Example:
# include<stdio.h>
# include<conio.h>
void main()
{
int i = 0;
while (i < 10)
{
printf("%d\n", i);
i++;
}
}
do—while loop
do—while loop is similar to a while loop, but it is guaranteed to execute the code in the loop at least once.
Syntax:
do
{
statement;
}
while(condition);
Example:
# include<stdio.h>
# include<conio.h>
void main()
{
int i = 0;
do
{
printf("%d\n", i);
i++;
}
while (i < 10);
}
Nested for loop
you can also use loops inside of other loops to create more complex patterns.
Syntax:
for(initialization, condition, incre/decre)
{
for(initialization, condition, incre/decre)
{
statement;
}
}
Example:
# include<stdio.h>
# include<conio.h>
void main()
{
int i;
for (int i = 0; i < 10; i++)
{
for (int j = 0; j < 10; j++)
{
printf("%d, %d\n", i, j);
}
}
}
Operators in C language
In the C programming language, operators are special symbols that perform specific operations on one or more operands and produce a result. Some common operators in C include:
- Arithmetic Operator
- Assignment Operator
- Comparison Operator
- Logical Operator
- Bitwise Operator
- Ternary Operator
- Relational Operator
- Miscellaneous Operator
Arithmetic Operator
Arithmetic Operators are +, -, *, /, %, ++, –. For example:
# include<stdio.h>
# include<conio.h>
void main()
{
int a = 5;
int b = 2;
int c = a + b; // c will be 7
int d = a - b; // d will be 3
int e = a * b; // e will be 10
int f = a / b; // f will be 2
int g = a%b; //g will be 1
}
Assignment Operator
Assignment operators are =, +=, -=, *=, /=, %=. For example:
# include<stdio.h>
# include<conio.h>
void main()
{
int a = 5; // assigns the value 5 to the variable a
a += 2; // equivalent to a = a + 2
a -= 2; // equivalent to a = a - 2
a *= 2; // equivalent to a = a * 2
a /= 2; // equivalent to a = a / 2
}
Comparison Operator
comparison operators are ==, <=, >=.
# include<stdio.h>
# include<conio.h>
void main()
{
int a = 5;
int b = 2;
if (a == b)
{
// this code will not be executed
}
if (a > b)
{
// this code will be executed
}
if (a < b)
{
// this code will not be executed
}
}
Logical Operator
Logical operators are &&, ||. For example:
# include<stdio.h>
# include<conio.h>
void main()
{
int a = 5;
int b = 2;
int c = 3;
if (a > b && a > c)
{
// this code will be executed because both conditions are true
}
if (a > b || a > c)
{
// this code will also be executed because at least one of the conditions is true
}
}
Bitwise Operator
Bitwise operators are &, /, XOR, <<, >>, >>>. For example:
# include<stdio.h>
# include<conio.h>
void main()
{
int a = 5; //
int b = 3; //
int c = a & b; // c will be 1 (binary representation is 001)
int d = a | b; // d will be 7 (binary representation is 111)
int e = a ^ b; // e will be 6 (binary representation is 110)
}
Ternary Operator
Ternary operators are:, ?. For example:
# include<stdio.h>
# include<conio.h>
void main()
{
int a = 5;
int b = 2;
int c = (a > b) ? a : b; // c will be 5 because the condition (a > b) is true
}
Relational Operator
Relational operators are <, >, <=, >=, ==, !=. For example:
# include<stdio.h>
# include<conio.h>
void main()
{
int a = 5;
int b = 2;
if (a == b)
{
// this code will not be executed because a is not equal to b
}
if (a > b)
{
// this code will be executed because a is greater than b
}
if (a < b) {
// this code will not be executed because a is not less than b
}
}
Miscellaneous Operator
Miscellaneous operators are &, *, ?. For example:
# include<stdio.h>
# include<conio.h>
void main()
{
int a = 5;
int *p = &a; // p is a pointer to the variable a
*p = 7; // this sets the value of a to 7 because *p is equivalent to a
}