Table of Contents
ToggleHello everyone welcome again to my new article in which I am going to explain some basic PHP and its programs with their output.
With the help of these PHP programs, you can easily understand the working and syntax of this programming language.
PHP Programming
PHP (Hypertext Preprocessor) is a programming language that is used for web development. It is a server-side scripting language, which means that it is executed on the server and then results in HTML being sent to the client.
this language is often used to build dynamic web pages. Dynamic web pages are pages that display different content every time they are accessed.
How to create a PHP server in Linux
For creating its server in Linux you have to follow these steps: –
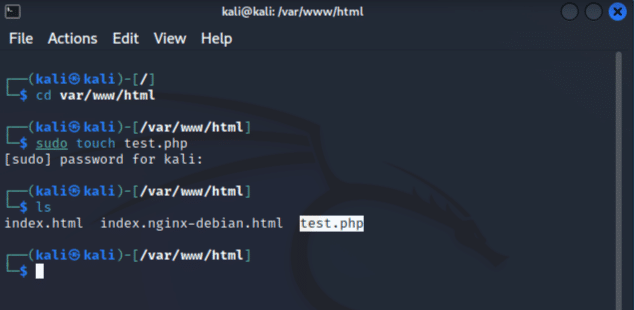
- Navigate to the directory: –
var/www/html/
- Create a PHP file in the HTML directory
- Write code in that file
- Go to your browser
- You can access the files in the HTML directory by: –
localhost/filename.php
Create a file by following these steps if you are not an administrator: –
- Open the terminal and navigate to
/home/cipherss/public_html/html/
directory - And then run this command: –
sudo touch filename.php
Basic PHP Programs
- Print “HELLO WORLD”
- Checking leap year
- Print table of number
- Print the Fibonacci series of number
- Print a Factorial of a number
- Check Armstrong number
- Check Palindrome number
- Print the reverse of the number
- Swapping of 2 numbers
- Find the area of given 2D shapes
Write a program to print “HELLO WORLD”
<?php
$a = "HELLO WORLD";
echo $a;
?>
//output in browser
HELLO WORLD
Write a program to check leap year
<?ph
$year = 2020;
if($year % 4 == 0)
{
echo $year .""."is a Leap Year.";
}
else
{
echo "$year is not a Leap Year.";
}
?>
//output in browser
2020 is a Leap Year.
Write a program to print a table of 7
<?php
$a = 7;
echo "Table of 7 is: <br>";
echo "<br>";
for($i=1; $i<=10; $i++)
{
$b = $i*$a;
echo "$a * $i = $b";
echo '<br>';
}
?>
//output in browser
Table of 7
7 * 1 = 7
7 * 2 = 14
7 * 3 = 21
7 * 4 = 28
7 * 5 = 35
7 * 6 = 42
7 * 7 = 49
7 * 8 = 56
7 * 9 = 63
7 * 10 = 70
Write a program to print the Fibonacci series of number
<?php
$num = 0;
$n1 = 0;
$n2 = 1;
echo "Fibonacci series for first 8 numbers: -";
echo "<br>";
echo $n1.' '.$n2.' ';
while ($num < 10 )
{
$n3 = $n2 + $n1;
echo $n3.' ';
$n1 = $n2;
$n2 = $n3;
$num = $num + 1;
}
?>
//output in browser
Fibonacci series for first 8 numbers: -
0 1 1 2 3 5 8 13 21 34 55 89
Write a program to print a Factorial of 5
<?ph
$num = 5;
$fact = 1;
for ($x=$num; $x>=1; $x--)
{
$fact = $fact * $x;
}
echo "Factorial of $num is: -" .$fact;
?>
//output in browser
Factorial of 5 is: - 120
Write a program to check Armstrong’s number
<?php
$num = 407;
$sum = 0;
$i = $num;
while($i!=0)
{
$x=$i%10;
$sum=$sum+$x*$x*$x;
$i=$i/10;
}
if($num==$sum)
{
echo $num." is an Armstrong Number.";
}
else
{
echo $n." is not an Armstrong Number.";
}
?>
//output in browser
407 is an Armstrong Number.
.
Write a program to check the Palindrome number
<?php
$num = 12321;
$i = 0;
$n = $num;
while(floor($num))
{
$mod = $num%10;
$i = $i * 10 + $mod;
$num = $num/10;
}
if($n==$x)
{
echo $n." is a Palindrome number.";
}
else
{
echo $n." is not a Palindrome number.";
}
?>
//output in browser
12321 is not a Palindrome number.
.
Write a program to print the reverse of the number
<?php
$num = 12345;
$rev = 0;
while ($num > 1)
{
$rem = $num % 10;
$rev = ($rev * 10) + $rem;
$num = ($num / 10);
}
echo "Reverse number of 12345 is " .$rev;
?>
//output in browser
Reverse number of 12345 is 54321
Write a program for swapping 2 numbers
Using the third variable
<?php
$a = 40;
$b = 50;
$c = $a;
$a = $b;
$b = $c;
echo "After swapping: -<br>";
echo "a =".$a." b=".$b;
?>
//output in browser
After swapping: -
a = 50 b = 40
Without using a third variable
<?php
$a = 40;
$b = 50;
$a = $a + $b;
$b = $a - $b;
$a = $a - $b;
echo "After swapping: <br>";
echo "Value of a: - " .$a ."</br>";
echo "Value of b: - " .$b ."</br>";
?>
//output in browser
After swapping: -
Value of a: - 50
Value of b: - 40
Write a program to find the area of given 2D shapes
Area of circle
<?php
$r = 2;
$area = 3.14 * $r * $r;
echo "Area of circle is: -" .$area;
?>
//output in browser
Area of circle is: - 12.56
Area of rectangle
<?php
$l = 10;
$b = 20;
$area = $l * $b;
echo "Area of rectange is: - " .$area;
?>
//output in browser
Area of rectangle is: - 200
Area of triangle
<?php
$l = 10;
$b = 20;
$area = 1/2 * $l * $b;
echo "Area of triangle is: - ".$area;
?>
//output in browser
Area of triangle is: - 100
Area of square
<?php
$a = 10;
$area = $a * $a;
echo "Area of square is: - ".$area;
?>
//output in browser
Area of square is: - 100